Exploring the Object Nature of JavaScript: A Deep Dive
Written on
Chapter 1: Introduction to JavaScript Objects
Have you ever wondered if it's true that nearly everything in JavaScript is treated as an object? Let’s delve deeper into this concept.
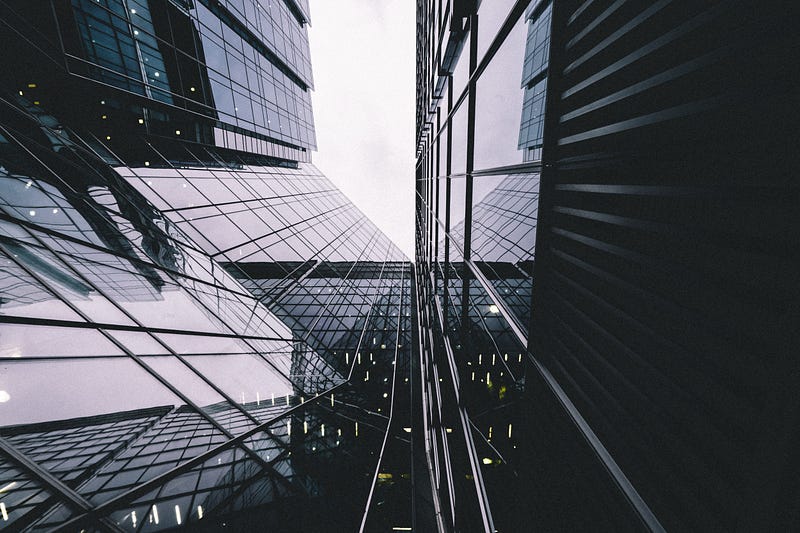
First, it's crucial to grasp what an object is in JavaScript. An object is essentially a mutable collection of properties, along with a hidden reference to its prototype. This definition might seem different from other programming languages where an object is often an instance of a class. In JavaScript, however, it is simply a collection of key-value pairs.
Once an object is instantiated, we have the ability to add, modify, or remove its properties. For example, consider this empty object:
const game = {};
game.name = 'Candy Crush'; // Property added
game.name = 'Candy Crush Saga'; // Property modified
delete game.name; // Property removed
Objects also possess a concealed property called __proto__, which links them to the prototype object. This means that all objects inherit properties from the built-in Object.prototype. You can verify this with the following code:
game.__proto__ === Object.prototype; // true
Object.getPrototypeOf(game) === Object.prototype; // true
Utilizing Object.getPrototypeOf() is the recommended method for determining an object's prototype.
Section 1.1: Understanding Arrays as Objects
Are arrays considered objects in JavaScript? Remember, an object is a mutable collection of properties. Let’s create an empty array and add some properties:
const flightArr = [];
flightArr.no = 815; // Property added
flightArr.from = 'Sydney'; // Property added
delete flightArr.no; // Property removed
We can then confirm that arrays have the hidden property linking them to their prototype, Array.prototype:
flightArr.__proto__ === Array.prototype; // true
Object.getPrototypeOf(flightArr) === Array.prototype; // true
While arrays are indeed objects, they should not be manipulated in the same manner as shown previously. Here is a proper way to create an array:
const arr = ['Jack', 'Locke', 'Kate'];
JavaScript emulates arrays using objects, where indices are treated as string keys. This means that accessing an element via string keys and numeric indices yields the same result:
arr['1']; // 'Locke'
arr[1] === arr['1']; // true
When using the typeof operator, JavaScript confirms that arrays are a type of object:
const arr = [];
typeof arr; // 'object'
Array.isArray(arr); // true
Section 1.2: Functions as Objects
Now, let’s explore whether functions qualify as objects. We can define a function and add properties to it:
const movie = function() {};
movie.title = 'Toc Toc'; // Property added
movie.year = 2017; // Property added
delete movie.year; // Property removed
Consider the Integer function that converts values into integers:
const Integer = function(value) {
return parseInt(value);
};
Integer('123'); // 123
We can also attach properties to this function, such as a maximum value or another function, and call the isInteger method:
Integer.MAX_VALUE = 9007199254740991;
Integer.isInteger = function(value) {
return Number.isInteger(value);
};
Just like built-in functions such as Number, String, and Boolean, our custom functions also act like objects. For instance:
const x = function() { return 'Hi'; };
x.type = 'Greeting';
console.log(x()); // 'Hi'
console.log(x.type); // 'Greeting'
Section 1.3: The Nature of Primitives
The built-in primitive types include boolean, number, bigint, string, symbol, null, and undefined. However, can we treat primitives as objects? Let's consider:
const text = "Hi";
text.type = 'greeting'; // Attempting to add a property
console.log(text.type); // undefined
Primitives cannot have properties added, altered, or removed. However, they can access methods from their respective prototype, appearing as if they are objects. When accessing properties, JavaScript temporarily converts the primitive to a corresponding object:
'in bruges'.toUpperCase(); // "IN BRUGES"
JavaScript constructs a temporary wrapper object to access properties. However, this does not apply to null and undefined, which remain as primitives and cannot have properties:
null.msg; // Uncaught TypeError
undefined.length; // Uncaught TypeError
Final Thoughts
In conclusion, while functions and arrays are indeed objects in JavaScript, not all values fit this description. Primitives are not objects but are treated as such to some extent. The exceptions to this rule are null and undefined, which are distinctly not treated as objects. Ultimately, aside from primitives, JavaScript treats nearly everything as an object.
To enhance your understanding, consider watching these informative videos:
This video delves into the concept that in JavaScript, everything behaves like an object.
Explore the question of whether everything in JavaScript is an object in this engaging video.