Creating and Reading QR Codes with Python: A Comprehensive Guide
Written on
Chapter 1: Introduction to QR Codes
In this guide, we will explore how to generate QR Codes using Python. QR Codes have become a staple in modern digital transactions, allowing for quick and efficient data sharing.
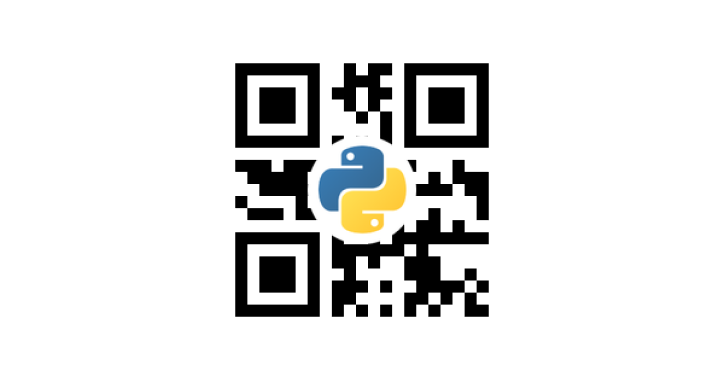
What is a QR Code?
QR Code stands for "Quick Response Code," a type of two-dimensional barcode designed for rapid scanning. Developed by Denso Wave in 1994, QR Codes have evolved to support seamless cardless transactions, becoming ubiquitous in digital payment methods. Understanding the functioning of QR Codes is essential for leveraging their benefits in various applications.
QR Codes can be classified into two main categories: Static and Dynamic.
Getting Started with QR Code Generation
Basic Setup
To begin generating QR Codes, you need to install the necessary library. Use the following command:
pip install qrcode
Next, import the library in your script:
import qrcode
Here’s a simple example to create your first QR Code:
img = qrcode.make("Asep Saputra")
img.save("qrcode1.png")
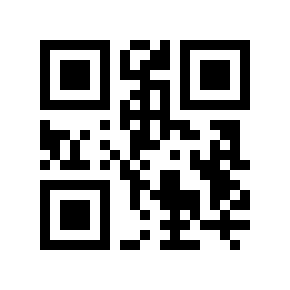
Using the make function is the easiest way to create a QR Code, as it automatically sets the version and fit parameters.
Customizing Your QR Code
In the next section, we will delve into customizing your QR Code's appearance. This includes changing its color, version, error correction level, box size, border width, and background color:
qr = qrcode.QRCode(
version=1,
error_correction=qrcode.constants.ERROR_CORRECT_L,
box_size=10,
border=4
)
qr.add_data('Some data')
qr.make(fit=True)
img = qr.make_image(fill_color="black", back_color="red")
img.save("qrcode2.png")
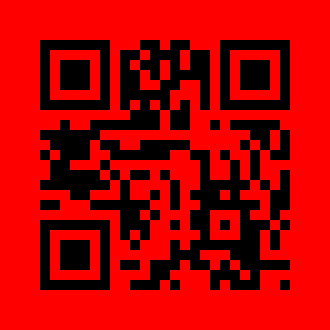
You can also define colors using RGB tuples:
img = qr.make_image(back_color=(255, 195, 235), fill_color=(100, 95, 35))
img.save("qrcode2-color.png")
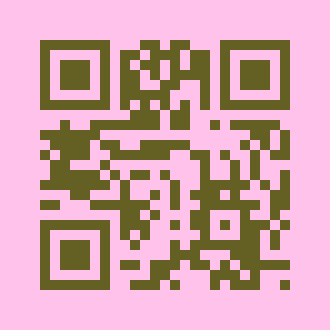
Error Correction Levels
- ERROR_CORRECT_L: Can correct up to 7% of errors.
- ERROR_CORRECT_M: Can correct up to 15% of errors.
- ERROR_CORRECT_Q: Can correct up to 25% of errors.
- ERROR_CORRECT_H: Can correct up to 30% of errors.
Advanced QR Code Features
For more advanced styling, you can use the StyledPilImage class to create rounded QR Codes:
from qrcode.image.styledpil import StyledPilImage
from qrcode.image.styles.moduledrawers import RoundedModuleDrawer
from qrcode.image.styles.colormasks import RadialGradiantColorMask
qr = qrcode.QRCode(error_correction=qrcode.constants.ERROR_CORRECT_L)
qr.add_data('Some data')
img_1 = qr.make_image(image_factory=StyledPilImage, module_drawer=RoundedModuleDrawer())
img_1.save("qrcode3.png")
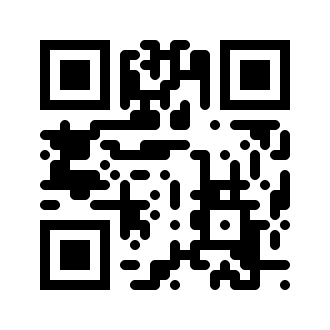
You can also apply a Radial Gradient Color Mask:
img_2 = qr.make_image(image_factory=StyledPilImage, color_mask=RadialGradiantColorMask())
img_2.save("qrcode4.png")
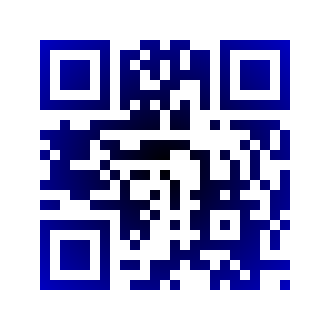
Additionally, you can embed an image in the center of the QR Code:
img_3 = qr.make_image(image_factory=StyledPilImage, embeded_image_path="python.png")
img_3.save("qrcode5.png")
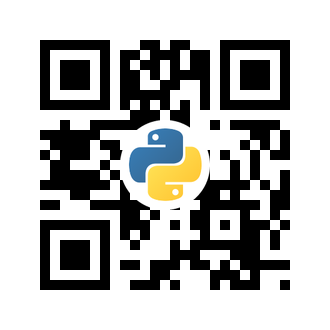
Chapter 2: Reading QR Codes
In this chapter, we will cover how to read QR Codes using OpenCV in Python.
This video tutorial titled "Generate A QR Code Using Python In 5 minutes" provides a quick overview of the QR Code generation process.
The second video, "Generate and Access QR Code Easily Using Python," offers detailed insights into working with QR Codes in Python.
Conclusion
Creating and reading QR Codes is a valuable skill in the digital age. I encourage you to explore these functionalities further. For those interested in ongoing learning, consider joining Medium Membership to support writers and access exclusive content.
References
For a more in-depth understanding of creating and reading QR Codes using Python, check out the article on Towards Data Science.