Choosing the Optimal Data Structure: Avoiding Arrays
Written on
Understanding Data Structures
When embarking on a programming project, it's crucial to evaluate the benefits and drawbacks of different data structures before making a decision. Relying solely on arrays can often lead to suboptimal outcomes.
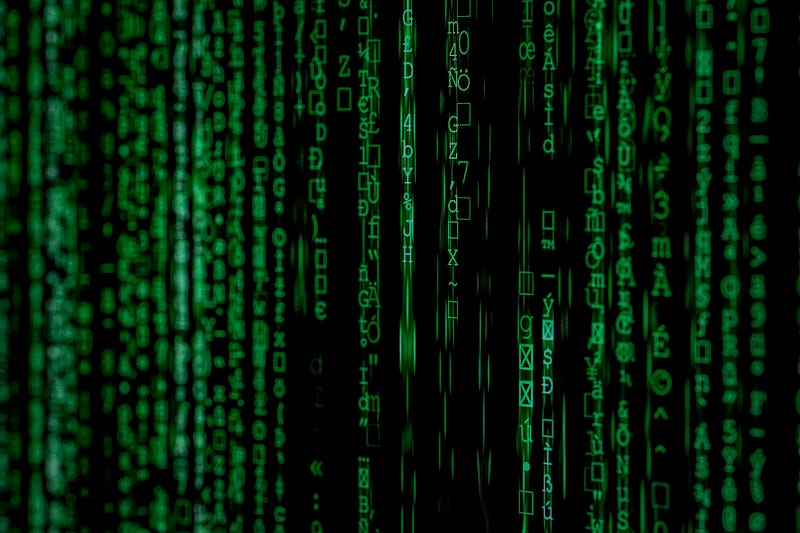
My background includes years spent at a physics research institute, where I transitioned into the scientific software sector. Many scientists at this institute had to create their own software for tasks such as data analysis or managing laboratory equipment. However, despite their expertise in science, they often lacked experience in professional software development. Most learned to program independently, frequently through online boot camps, which provided only a foundational understanding. Consequently, the code produced was often difficult to maintain, hard to read, and suffered from performance issues.
To address these challenges, I began offering hands-on courses aimed at helping Ph.D. students avoid common pitfalls. While I don’t consider myself the best software developer, it’s essential for anyone writing code—whether they aim to be professionals or not—to grasp certain fundamental concepts. One critical aspect that tends to be overlooked in basic boot camps is the selection of the appropriate data structure.
The Importance of Data Structure Selection
In my courses, I observed that students would instinctively gravitate toward arrays when managing collections of objects. Many were unaware of alternative container types, and even those who were often overlooked them. However, arrays frequently represent the wrong choice. Consequently, I provided an overview of the pros and cons associated with common container types, demonstrating their appropriate applications.
Given that this information proved beneficial for my students, it may also be useful to others. For the purpose of this discussion, I will focus on Python and its standard library, though these principles are applicable across various programming languages.
Arrays: The Basic Container Type
The array is typically the first container type encountered by those learning a programming language. It consists of a contiguous block of memory where each item occupies the same amount of space.

Arrays allow for quick access to specific items via their indices, as the computer knows the starting memory address of the array and can calculate the position of the k-th item by adding k times the size of a single item to that address.
While this efficiency is advantageous, there are notable drawbacks. Arrays can become problematic when frequent structural modifications are necessary. For example, inserting an item at the beginning of an array requires increasing the memory allocation and shifting all existing items, leading to considerable overhead.
Another crucial factor is readability. When the objects stored in a container have a natural order, like time-stamped data, array notation may be adequate. However, for unordered data—such as a collection of RGB color codes—using array indices is not intuitive. Instead of color_codes[3], wouldn’t it be more understandable to use color_codes['RED']? This leads us to the next data structure: dictionaries.
Dictionaries: Key-Value Pairs
In Python, dictionaries are another fundamental container type. They are known by various names in different programming languages, such as hash maps in Java or associative arrays. The distinguishing feature of dictionaries is that they allow access and storage of data items (values) via named keys rather than numerical indices.
For example, in Python, you can define color codes as follows:
color_codes = {
'RED': '#FF0000',
'GREEN': '#00FF00',
'BLUE': '#0000FF'
}
This enables more readable code, as you can access items through their keys, like color_codes['RED']. Instead of being stored in a contiguous block, dictionary items are typically distributed across memory. The advantage of this structure is that when you add a new item, the computer simply allocates memory at the location corresponding to the new key, avoiding the need for copy operations.
However, dictionaries come with their own set of disadvantages. Accessing a single item is fast, but retrieving multiple items can be slow due to their scattered nature in memory, which can hinder performance in computation-heavy applications.
Choosing the right data structure in Coding Interviews - YouTube
Sets: Enforcing Uniqueness
While both arrays and dictionaries allow duplicate entries, sets offer a solution for maintaining uniqueness. In Python, adding duplicates to a set does not alter its content.
For instance:
s = set()
s.add('Apple')
s.add('Orange')
s.add('Apple')
print(s)
This will output:
{'Orange', 'Apple'}
Sets also support standard mathematical operations like union and intersection. However, like dictionaries, sets are unordered, meaning the order of elements during iteration is not guaranteed.
Linked Lists: A Less Common Choice
A linked list is another type of data structure, though it is not part of the standard Python library. Unlike Python's dynamic arrays, a true linked list consists of elements that contain both the data and a pointer to the next element.

To access a specific element, the computer must traverse the list from the beginning, which can be slow. However, inserting an element is relatively quick, as it only requires updating pointers rather than moving existing elements.
While linked lists have their uses, they are often less favorable compared to dictionaries because they lack the advantages of fast access.
Other Data Structures: Trees, Queues, and More
Numerous additional container data structures, such as trees, queues, and stacks, offer unique strengths. Trees, for example, excel at performing rapid searches or organizing elements effectively.
I plan to cover these data structures in more detail in a follow-up article, so if this topic interests you, stay tuned.
Thank you for reading!
For more insights, visit plainenglish.io. Consider subscribing to our free weekly newsletter for exclusive writing opportunities and advice within our community Discord.
Array vs Linked List - Pros & Cons | Which data structure is better & when? | DSA - YouTube