Avoid These 6 Mistakes to Enhance Your Code Quality
Written on
Chapter 1: Introduction to Code Quality Mistakes
We've all invested countless hours on projects that, despite functioning correctly, end up looking chaotic. Before poor coding habits take hold, it's crucial to recognize these six significant mistakes that can deteriorate your code quality.
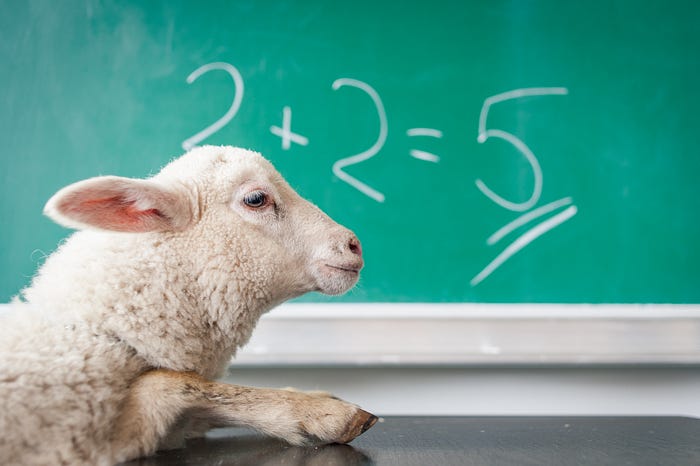
Section 1.1: Common Mistake 1 - Overly Complex Functions
What’s wrong with this Python code snippet?
def validate(name, email):
if re.fullmatch(name_regex, name):
print("valid name")else:
print("invalid name")if re.fullmatch(email_regex, email):
print("valid email")else:
print("invalid email")
Although we have imported the necessary regular expression module and defined all required variables, this function is too lengthy. Ideally, each function should focus on a single task. Our current function validates both the name and email, performing two separate actions.
To enhance readability, we could split this into two distinct functions:
def validate_email(email):
if re.fullmatch(email_regex, email):
print("valid email")else:
print("invalid email")
def validate_name(name):
if re.fullmatch(name_regex, name):
print("valid name")else:
print("invalid name")
Why is this important? Shorter functions are easier to understand, write, and test.
Section 1.2: Common Mistake 2 - Ambiguous Naming
Creative naming can sometimes backfire. Consider this pseudocode:
flavors = [vanilla, chocolate, raspberry]
victim = flavors.pop(1)
Or this less imaginative example:
array = [vanilla, chocolate, raspberry]
element = array.pop(1)
In both instances, the names chosen fail to enhance code quality and are generally poor programming practices. Names should be both descriptive and concise.
Section 1.3: Common Mistake 3 - Lack of Comments
Even if you can't be overly creative with variable names, don't leave your code silent. Comments are vital; they clarify what each part of your code does, aiding both others and your future self in understanding and modifying it without excessive effort.
Section 1.4: Common Mistake 4 - Repetition
Imagine writing a book that repeats the same chapter multiple times—your reader would be frustrated! The same principle applies to coding. Stick to the "Don't Repeat Yourself" (DRY) principle. If you find yourself copying and pasting code, it's a sign you should refactor that code into a function.
Section 1.5: Common Mistake 5 - Inconsistent Formatting
Consider this pseudocode:
if (time < 7 AM) {
snooze
}
else if (time >= 7 AM)
{
wake up
}
While different bracket placements may not affect the code's functionality, inconsistency can lead to confusion. Consistency in formatting is crucial in programming.
Section 1.6: Common Mistake 6 - Excessive Global Variables
Global variables, defined outside of functions, are accessible throughout the program. If they are not constant, they can be modified by different functions, potentially leading to program failures. Instead of the following:
flavors = [vanilla, chocolate, raspberry]
def replace_flavor():
flavors.pop()
flavors.append("pistachio")
Consider using local variables:
def replace_last_flavor():
flavors = [vanilla, chocolate, raspberry]
flavors.pop()
flavors.append("pistachio")
Chapter 2: Importance of Planning
This video, titled "5 Mistakes That Are Killing Your Coding Progress," highlights common pitfalls in coding practices.
The second video, "Mistakes I Made as an Intermediate Learner," discusses self-sabotage and poor resource selection in coding.
In summary, here are the key takeaways for those in a hurry:
- Avoid overly complex functions
- Use clear and descriptive names
- Incorporate comments as necessary
- Steer clear of code repetition
- Maintain consistent formatting
- Limit the use of global variables
- Plan your code effectively (and review it as needed)
Thank you for taking the time to read this!