Enhancing React Applications with Victory Charts: A Complete Guide
Written on
Chapter 1: Introduction to Victory Charts
In this article, we will explore how to incorporate dynamic charts into our React applications using the Victory library. This powerful tool allows for effective data visualization, making it easier to present complex information.
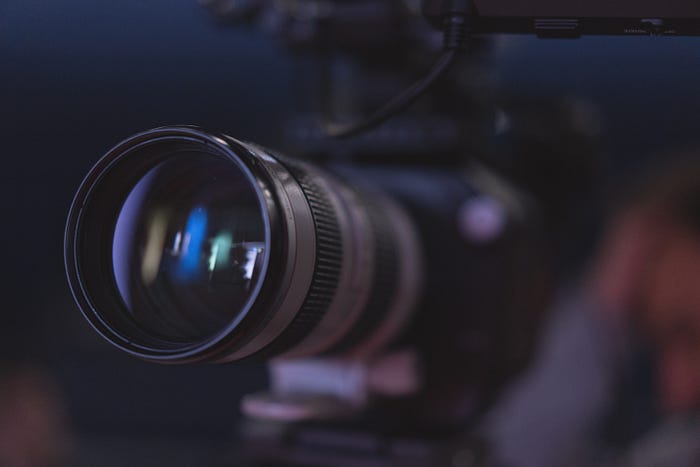
Section 1.1: Implementing Transitions
The VictoryBar component enables us to apply transition effects to animations. For example, we can use the following code:
import React, { useEffect, useState } from "react";
import { VictoryBar, VictoryChart } from "victory";
const generateRandomNumber = (min, max) => Math.floor(min + Math.random() * max);
const createDataSet = () => {
const numberOfBars = generateRandomNumber(6, 10);
return Array(numberOfBars)
.fill()
.map((_, index) => {
return {
x: index + 1,
y: generateRandomNumber(2, 100)
};
});
};
export default function App() {
const [data, setData] = useState([]);
useEffect(() => {
const interval = setInterval(() => {
setData(createDataSet());}, 3000);
return () => clearInterval(interval);
}, []);
return (
<VictoryChart>
<VictoryBar
data={data}
animate={{
onExit: { duration: 500 },
duration: 1000
}}
style={{ data: { fill: "orange" } }}
/>
</VictoryChart>
);
}
In this code, we generate random data for our chart and refresh it every 3 seconds. By using the animate prop in the VictoryBar component, we can add visual transition effects for the bars.
Section 1.2: Enabling Brush and Zoom Features
To incorporate brush and zoom functionalities into our charts, we can utilize the containerComponent prop of VictoryChart. Below is an example:
import React from "react";
import { VictoryChart, VictoryScatter, VictoryZoomContainer } from "victory";
const generateRandomNumber = (min, max) => Math.floor(min + Math.random() * max);
const createDataSet = () => {
return Array(50)
.fill()
.map(() => {
return {
x: generateRandomNumber(1, 50),
y: generateRandomNumber(10, 90),
size: generateRandomNumber(8) + 3
};
});
};
export default function App() {
return (
<VictoryChart containerComponent={<VictoryZoomContainer />}>
<VictoryScatter
data={createDataSet()}
style={{
data: { fill: ({ datum }) => (datum.y % 5 === 0 ? "tomato" : "black") }}}
/>
</VictoryChart>
);
}
Here, we set the containerComponent to the VictoryZoomContainer, allowing users to interactively zoom in and out on the chart data.
Chapter 2: Creating Interactive Charts
The first video, "Animated Bar Charts with Expo (React Native), Victory Native, and Skia," provides a visual guide on how to create animated bar charts using Victory.
The second video, "Animated Line Charts with Expo (React Native), Victory Native, and Skia," showcases the process of developing animated line charts with the same technologies.
Section 2.1: Handling Zoom and Brush Domains
We can further enhance our charts by managing zoom and brush domains. Here's an example:
import React, { useState } from "react";
import { VictoryAxis, VictoryBrushContainer, VictoryChart, VictoryLine, VictoryZoomContainer } from "victory";
export default function App() {
const [selectedDomain, setSelectedDomain] = useState();
const [zoomDomain, setZoomDomain] = useState();
const onZoom = (domain) => {
setSelectedDomain(domain);};
const onBrush = (domain) => {
setZoomDomain(domain);};
return (
<>
<VictoryChart containerComponent={<VictoryBrushContainer brushDomain={selectedDomain} onBrushDomainChange={onBrush} />}>
<VictoryLine /></VictoryChart>
<VictoryChart containerComponent={<VictoryZoomContainer zoomDomain={zoomDomain} onZoomDomainChange={onZoom} />}>
<VictoryLine /></VictoryChart>
</>
);
}
In this example, we manage the zoom and brush domains for two separate VictoryCharts, allowing users to interact and observe data changes seamlessly.
Conclusion
By integrating transitions, brush, and zoom functionalities, we can significantly enhance the interactivity of charts in our React applications using Victory. This flexibility allows developers to create engaging and informative data visualizations.