Maximize Performance with Stackalloc: Day 28 of .NET Challenge
Written on
Chapter 1: Introduction to Stackalloc
On Day 28 of our 30-Day .NET Challenge, we dive into the benefits of using stackalloc for performance optimization in C#. .NET applications depend on a Garbage Collector (GC) for managing memory allocation and deallocation, which simplifies development but can lead to inefficiencies if not handled properly. This article explores how stackalloc can improve application performance.
Learning Objectives:
- Grasp the concept of stackalloc
- Identify issues with conventional heap allocation
- Understand optimal scenarios for stackalloc usage
Prerequisites for Developers:
- Basic knowledge of C# programming
- Familiarity with for loops
Section 1.1: What is Stackalloc?
Stackalloc is a keyword in C# that allows memory allocation on the stack, as opposed to the heap, which is managed by the Garbage Collector. Memory allocated with stackalloc is automatically released once the method completes.
Section 1.2: Challenges with Traditional Heap Allocation
To illustrate the drawbacks of heap allocation, consider the following example where memory for a double array is allocated on the heap.
private double CalculateSum(double[] values)
{
double sum = 0;
for (int i = 0; i < values.Length; i++)
{
sum += values[i];}
return sum;
}
If this method is invoked frequently, it adds significant overhead for garbage collection, ultimately reducing application performance.
Section 1.3: Optimal Use of Stackalloc
Here’s a revised version of the previous code that utilizes stackalloc:
private unsafe double CalculateSum(int count)
{
double sum = 0;
double* values = stackalloc double[count]; // Allocate memory on the stack
for (int i = 0; i < count; i++)
{
values[i] = SomeValue(i); // Assume SomeValue is a method returning a double
sum += values[i];
}
return sum;
}
In this version, we allocate memory on the stack, eliminating the need for garbage collection and enhancing execution speed, thereby reducing strain on the GC. For applications where performance is crucial, efficient memory management is vital, and stackalloc offers a way to achieve this.
Chapter 2: Complete Code Implementation
Create a class called StackAlloc and implement the following code:
public static class StackAlloc
{
static int count = 10000; // Number of elements
static double[] values = new double[count];
public static void BadWay()
{
FillValues(values);
// Calculate sum using heap allocation
double heapSum = CalculateSumHeap(values);
Console.WriteLine($"Heap allocation sum: {heapSum}");
}
public static void GoodWay()
{
FillValues(values);
// Calculate sum using stackalloc
double stackSum = CalculateSumStackalloc(count);
Console.WriteLine($"Stackalloc sum: {stackSum}");
}
private static void FillValues(double[] values)
{
for (int i = 0; i < values.Length; i++)
{
values[i] = SomeValue(i);}
}
private static double SomeValue(int i)
{
// Sample value function
return i * 2.5;
}
private static double CalculateSumHeap(double[] values)
{
double sum = 0;
for (int i = 0; i < values.Length; i++)
{
sum += values[i];}
return sum;
}
private static unsafe double CalculateSumStackalloc(int count)
{
double sum = 0;
double* values = stackalloc double[count];
for (int i = 0; i < count; i++)
{
values[i] = SomeValue(i);
sum += values[i];
}
return sum;
}
}
To execute this from the main method, use the following code:
#region Day 28: Use Stackalloc
static string ExecuteDay28()
{
StackAlloc.BadWay();
StackAlloc.GoodWay();
return "Executed Day 28 successfully..!!";
}
#endregion
The console output will be:
Heap allocation sum: 124987500
Stackalloc sum: 124987500
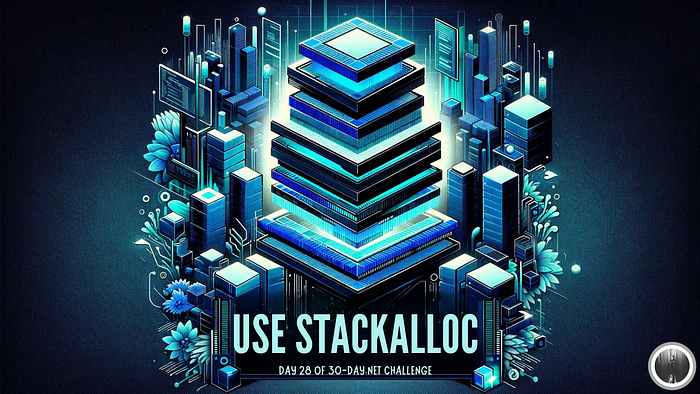
For more detailed code, visit our GitHub repository dedicated to C# Programming.
Thank you for joining the C# community! Before you go:
Follow us on: Youtube | X | LinkedIn | Dev.to
Explore our other resources: GitHub
Discover more content related to C# Programming.