Exciting Enhancements in Java 22: What Developers Need to Know
Written on
Chapter 1: Introduction to Java 22
On March 19th, Oracle unveiled Java 22, marking a significant update to one of the most widely-used programming languages in the world. This new version of the Oracle JDK introduces numerous enhancements aimed at improving performance, stability, and security. These updates are designed to help developers code more efficiently, innovate, and contribute to their organizations' growth. The modifications impact the Java language, its operational capabilities, and the tools available within the Java Development Kit (JDK). Let's explore three of the standout features included in this release.
Section 1.1: Unnamed Variables and Patterns
One notable improvement focuses on a common coding challenge: the declaration of variables that are not utilized in the program's logic. This often occurs in scenarios where the primary goal is to trigger side effects rather than to return a result, such as in exception handling. Developers frequently create variables in catch blocks that are never used, like this example:
try {
int number = Integer.parseInt(s);
} catch (NumberFormatException e) {
LOG.error("Bad number!");
}
With Java 22, you can simplify this by using an underscore (_) to denote such unused variables:
try {
int number = Integer.parseInt(s);
} catch (NumberFormatException _) {
LOG.error("Bad number!");
}
This feature, which was initially introduced in JDK 21 as a preview, is now a permanent aspect of Java with no alterations to its initial implementation.
Subsection 1.1.1: Visual Explanation of Unnamed Variables
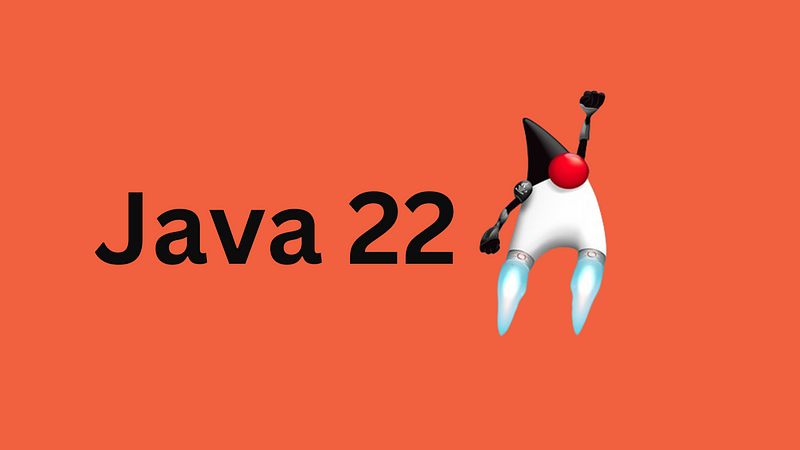
Section 1.2: Statements Before super(…) (Preview)
In Java, inheritance allows a subclass to take on properties and methods from its superclass, enhancing its functionality while also enabling the addition of new features. A crucial part of this inheritance model is the order of initialization, which mandates that a superclass's attributes must be initialized before any modifications can be made in the subclass.
This strict sequence often imposes limitations during object creation, as Java requires that any constructor must call its superclass's constructor first. For example, if we need to validate an argument passed to a superclass constructor, doing so straightforwardly was previously challenging. A common workaround looked like this:
public PositiveBigInteger(long value) {
super(value);
if (value <= 0)
throw new IllegalArgumentException("Not Positive!");
}
However, with the new feature, we can validate the argument more directly:
public PositiveBigInteger(long value) {
if (value <= 0)
throw new IllegalArgumentException("Not Positive!");
super(value);
}
Note that this feature is currently in preview mode in Java 22, allowing developers to test and provide feedback on its functionality, which may evolve in future updates.
Chapter 2: Stream Gatherers (Preview)
The introduction of the Stream API in Java 8 was revolutionary, enabling developers to process sequences of elements in a functional manner. Nevertheless, certain tasks remained cumbersome due to the limited set of operations available. To address this, Java 22 has introduced Stream::gather(Gatherer). This innovative feature allows developers to implement intermediate stream operations more flexibly, akin to how Stream::collect(Collector) facilitates the conclusion of stream processing.
The java.util.stream.Gatherers class presents several built-in gatherers, including fold, scan, and windowFixed, which enhance the Stream API with valuable new capabilities. For example, the fold gatherer is particularly useful for tasks that require order-sensitive reduction, producing a single result from the processed elements.
Here’s a practical illustration of how the fold gatherer can be utilized:
Optional<String> numberString =
Stream.of(1,2,3,4,5,6,7,8,9)
.gather(
Gatherers.fold(() -> "", (string, number) -> string + number))
.findFirst();
This would yield an Optional of "123456789". Keep in mind that this feature is also currently in preview mode in Java 22.
Section 2.1: Conclusion
These updates in Java 22 are truly exciting. I invite you to share your thoughts in the comments regarding which features you find most appealing. If you found this overview informative, consider following me for further insights and updates on Java.